Docs
Image Trail
Image Trail
Image Trail adds a dynamic, interactive trail of images that follows the cursor, enhancing user engagement and visual appeal on your website.
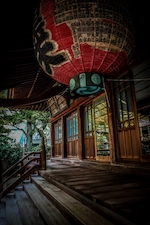
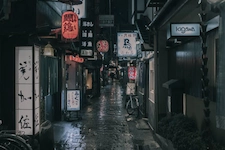
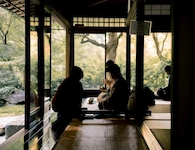
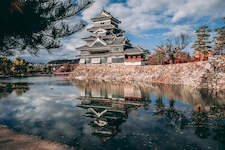
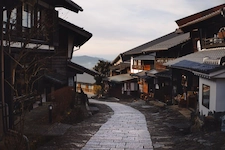
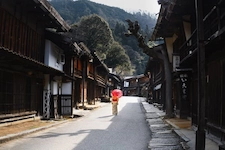
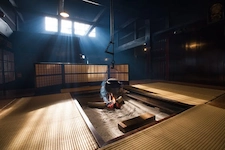
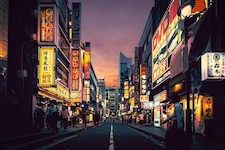
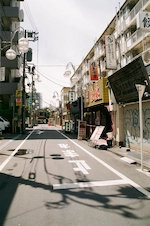
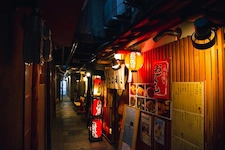
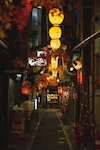
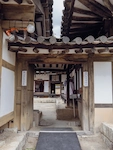
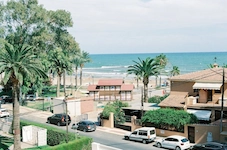
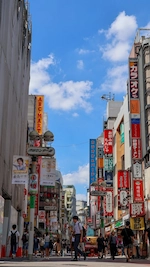
Hover Me
Installation
pnpm dlx shadcn@latest add to-setup
Usage
import { ImageTrail } from "@/components/ui/image-trail";
const imageUrls = [
"/images/components/image-trail/image-trail-1.webp",
"/images/components/image-trail/image-trail-2.webp",
"/images/components/image-trail/image-trail-3.webp",
"/images/components/image-trail/image-trail-4.webp",
"/images/components/image-trail/image-trail-5.webp",
];
<ImageTrail images={imageUrls} imageHeight={150} imageWidth={150} />
Props
ImageTrail
props.
Prop | Type | Description | Default |
---|---|---|---|
images | string[] | An array of image URLs to be used in the trail effect. | [] |
imageWidth | number | The width of each image in the trail (in pixels). | 200 |
imageHeight | number | The height of each image in the trail (in pixels). | 200 |
threshold | number | The minimum distance the mouse must move to trigger an image change. | 50 |
duration | number | The duration (in seconds) of the animation for each image. | 1.6 |