Docs
Expandable Card
Expandable Card
A versatile and engaging UI component that allows users to explore content in a more immersive way.
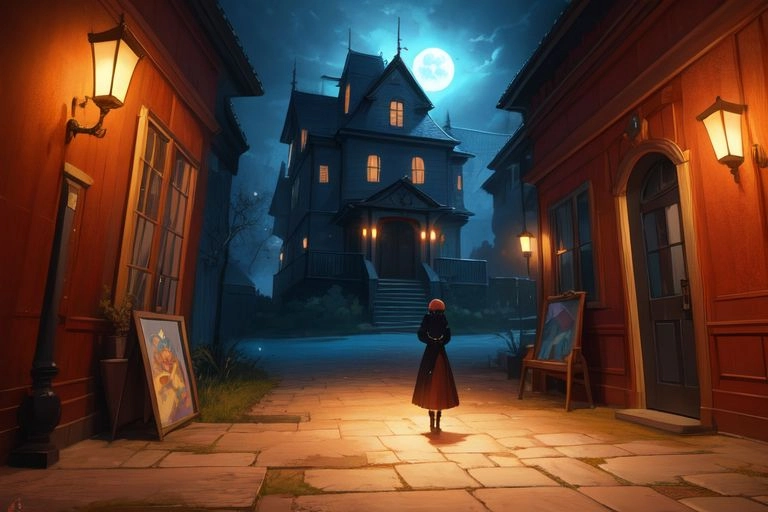
A Yokai Tale
Whispering Forest
Installation
Usage
import { ExpandableCard } from "@/components/ui/expandable-card";
<ExpandableCard
title="Whispering Forest"
src="/images/components/expandable-card/haunted-house.webp"
description="A Yokai Tale"
>
{content}
</ExpandableCard>
Props
ExpandableCard props.
Prop | Type | Description | Default |
---|---|---|---|
title | string | The title displayed on the card. | - |
src | string | The URL of the image displayed at the top of the card. | - |
description | string | A short description displayed below the title. | - |
children | React.ReactNode | Additional content displayed inside the expanded card. | - |
className | string | Custom CSS classes for styling the card. | - |
classNameExpanded | string | CSS classes applied when the card is expanded. | - |
[key: string] | any | Any additional props passed to the parent component. | - |