Docs
Cloud Orbit
Cloud Orbit
This component creates a dynamic and interactive experience, where each icon orbits in a fluid motion. Fully customizable, it's ideal for showcasing your tech stack or the tools your product uses.
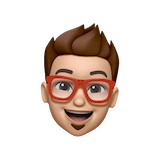







Installation
Usage
import { CloudOrbit, OrbitingImage } from "@/registry/components/cloud-orbit";
const orbitingImagesData = [
{
speed: 20,
radius: 119,
size: 53,
startAt: 0.15625,
images: [
{
name: "Deepseek Logo",
url: "/images/components/cloud-orbit/deepseek-logo.webp",
},
{
name: "Drizzle ORM Logo",
url: "/images/components/cloud-orbit/drizzle-orm-logo.webp",
},
],
},
...
];
<CloudOrbit
duration={3}
size={160}
images={[
{
name: "Charlie Avatar",
url: "/images/components/cloud-orbit/avatar-1.webp",
},
{
name: "Tommy Avatar",
url: "/images/components/cloud-orbit/avatar-2.webp",
},
]}
className=""
>
{orbitingImagesData.map((orbit, index) => (
<OrbitingImage
key={index}
speed={orbit.speed}
radius={orbit.radius}
size={orbit.size}
startAt={orbit.startAt}
images={orbit.images}
/>
))}
</CloudOrbit>
Placing the icons at the correct angle can be a bit tricky. For easier setup, use the positions from the demo and adjust them visually. If you need help, just reach out!
Props
cloud-orbit props.
Prop | Type | Description | Default |
---|---|---|---|
duration | number | Duration of the animation cycle in seconds. | 3 |
children | React.ReactNode | Child elements to be rendered inside the component. | - |
size | number | Size of the main orbit container in pixels. | 160 |
className | string | Additional custom classes for styling. | - |
images | Array<Object> | Array of image objects, each containing a url and name . | [] |
props | Record<string, any> | Additional HTML attributes for the component's wrapper div. | - |
orbiting-image props.
Prop | Type | Description | Default |
---|---|---|---|
speed | number | Speed of the orbiting motion (lower is slower). | 20 |
radius | number | Radius of the orbit path in pixels. | 100 |
startAt | number | Delay before animation starts, in seconds. | 0 |
size | number | Size of each orbiting image in pixels. | 80 |
className | string | Additional custom classes for styling. | - |
images | Array<Object> | Array of image objects, each containing a url and name . | [] |
duration | number | Duration of the animation cycle for each orbiting image. | 3 |
props | Record<string, any> | Additional HTML attributes for the component's wrapper div. | - |
Disclaimer
We are not affiliated with any of the brands whose logos are used in this component. These logos are displayed for demonstration purposes only.